Building a CI/CD Pipeline for Java Microservices with Jenkins, Docker, and Kubernetes
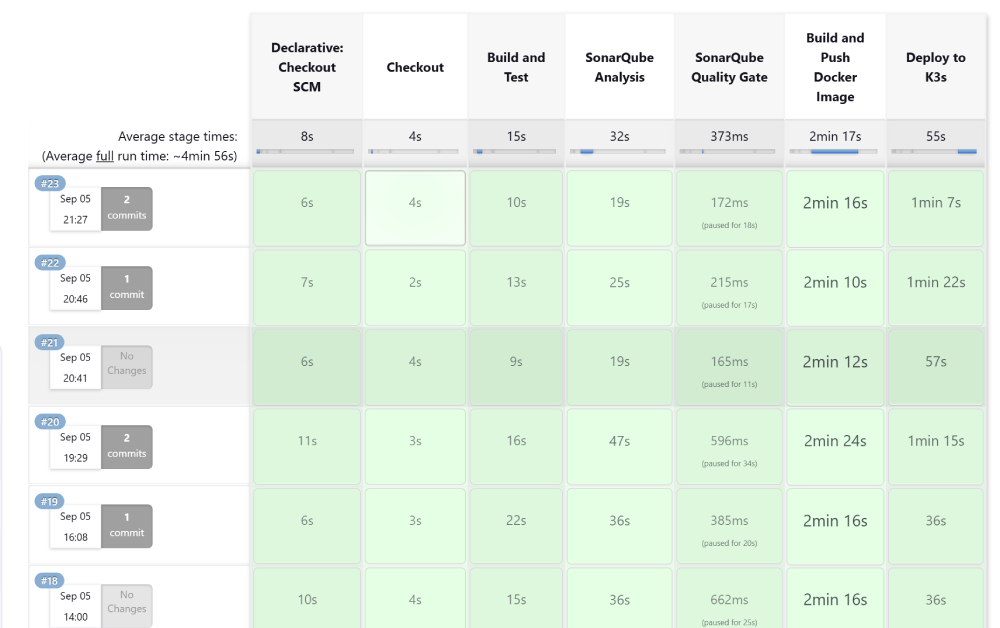
Setting up an automated CI/CD pipeline can significantly streamline your development process, especially for microservices. This guide walks you through implementing a CI/CD pipeline for a Java-based microservice using Jenkins, Docker, and Kubernetes (specifically, K3s). We’ll use Jenkins agents deployed in Kubernetes pods to enhance scalability and flexibility.
Fundamental Knowledge of CI/CD
1. What is CI/CD?
- Continuous Integration (CI): The practice of automatically testing and integrating code changes into a shared repository. It involves frequently merging code changes into the main branch, where automated builds and tests are run to detect issues as early as possible.
- Continuous Delivery (CD): The practice of automatically preparing code changes for production release. Continuous delivery ensures that the codebase is always in a deployable state, allowing for frequent and reliable deployments.
- Continuous Deployment: A step beyond continuous delivery, where code changes are automatically deployed to production without manual intervention, provided they pass all automated tests.
2. Key Concepts of CI/CD
- Build Automation: Automating the process of compiling source code into executable applications. Build automation tools (like Maven for Java) help streamline this process.
- Automated Testing: Running tests automatically during the CI/CD process to validate code changes. This includes unit tests, integration tests, and functional tests.
- Artifact Management: Storing and managing built artifacts (e.g., Docker images, JAR files) in a repository (like Docker Hub or Nexus) to ensure that the correct versions are used during deployment.
- Deployment Automation: Automating the deployment of applications to various environments (development, staging, production) using tools like Kubernetes, which handles scaling, load balancing, and self-healing.
3. Benefits of CI/CD
- Faster Time to Market: Automated processes enable teams to release features and updates more rapidly, improving overall competitiveness.
- Reduced Risk: Smaller, incremental changes minimize the impact of potential failures, making it easier to roll back changes if necessary.
- Higher Quality Code: Automated testing and quality checks lead to higher-quality code and a more stable product in production.
4. Best Practices for CI/CD
- Keep Builds Fast: Optimize the CI/CD pipeline to ensure builds and tests run quickly. This encourages frequent integrations.
- Test Early and Often: Run tests at various stages of the pipeline to catch issues early.
- Maintain a Clean and Readable Pipeline: Ensure that your Jenkinsfile (or equivalent configuration) is well-organized and easy to understand for better maintainability.
- Monitor and Measure: Use metrics to evaluate the performance of your CI/CD pipeline and identify areas for improvement.
Advantages of Using Jenkins with Docker and Kubernetes for CI/CD
1. Scalability
- Dynamic Agent Provisioning: By utilizing Jenkins agents as Kubernetes pods, you can dynamically provision build agents based on demand. This scalability allows you to handle fluctuating workloads efficiently, scaling up during peak times and scaling down during quieter periods.
- Resource Optimization: With Kubernetes, resources are allocated more effectively. Jenkins agents are created on-demand, meaning that you only use resources when necessary. This helps reduce costs and improves overall efficiency.
2. Isolation
- Environment Consistency: Each Jenkins job runs in its own isolated container. This ensures that dependencies, configurations, and environment settings do not conflict with one another. Each build is performed in a clean environment, eliminating “it works on my machine” issues.
- Security: Running builds in isolated containers reduces the risk of vulnerabilities impacting the host system. Each container has its own file system and processes, enhancing security.
3. Faster Feedback Loop
- Automated Testing: Integrating automated tests in the CI/CD pipeline means developers receive immediate feedback on their code. This helps in catching issues early in the development process, reducing the cost and effort required for later fixes.
- Continuous Integration: By automating the build and testing process, teams can integrate code changes more frequently (e.g., multiple times a day), which leads to faster releases and quicker iterations.
4. Streamlined Deployments
- Consistent Deployments: Using Docker images ensures that the same image is used across development, testing, and production environments. This consistency reduces deployment-related issues and enhances reliability.
- Blue-Green Deployments: Kubernetes supports deployment strategies such as blue-green deployments, allowing for safer updates with minimal downtime. If a new version has issues, it’s easy to roll back to the previous version.
5. Improved Collaboration
- Version Control Integration: CI/CD encourages developers to collaborate more effectively through version control systems. Pull requests can trigger automated builds and tests, facilitating better communication among team members.
- Feedback Mechanism: With automated pipelines, stakeholders can provide feedback earlier in the development process, enabling teams to make adjustments based on user or customer input.
6. Visibility and Monitoring
- Centralized Logging and Monitoring: Jenkins, in conjunction with Kubernetes, can be configured to send logs and metrics to centralized logging and monitoring systems. This helps teams track the health of their applications and pipelines, making it easier to identify and troubleshoot issues.
Prerequisites
Ensure you have the following set up in your environment:
- Jenkins installed and configured.
- K3s Kubernetes cluster with permissions for Jenkins to create and manage pods.
- Docker for containerization.
- Git repository with your microservice code.
- SonarQube for code quality analysis (optional but recommended).
Step 1: Overview of Jenkins Kubernetes Agents
The Jenkins Kubernetes Plugin allows Jenkins to dynamically create pods within Kubernetes (K3s in this case) to serve as agents for specific tasks. This provides isolated environments for each build, conserving resources and enhancing security. Each task is run in a separate container within the pod, which is removed after the job is complete, optimizing resource usage.
Step 2: Setting Up Jenkins and Kubernetes Integration
To start, configure Jenkins to work with K3s, enabling it to dynamically create pods as agents for specific builds.
1. Configure Kubernetes Cloud in Jenkins
- Manage Jenkins → Manage Nodes and Clouds → Configure Clouds.
- Select Add a new cloud and choose Kubernetes.
- Set the Kubernetes URL to your K3s cluster’s API URL.
- Kubernetes Namespace: Specify the namespace where Jenkins pods will be created.
- Credentials: Add Kubernetes credentials for Jenkins if you haven’t already. This should be a ServiceAccount with permissions to create and manage pods.
- Set Pod Retention to either
Always
orNever
, depending on whether you want the pod to be deleted after each build.
2. Configure the Jenkins Agent Pod Template
- Under Kubernetes Cloud Configuration, add a Pod Template.
- Name: Use
jenkins-agent
. - Labels: Assign
k8s-agent
(this will be referenced in yourJenkinsfile
). - Configure each container within the pod to handle specific tasks:
- jnlp: Required for Jenkins communication.
- maven: Handles the build process.
- docker: Builds and pushes Docker images.
- kubectl: Deploys to K3s.
Step 3: Writing the Jenkinsfile
With Jenkins and Kubernetes integrated, let’s set up a Jenkinsfile
to automate the CI/CD pipeline. Here’s an example pipeline for a Java microservice:
pipeline {
agent {
kubernetes {
yaml '''
apiVersion: v1
kind: Pod
metadata:
name: jenkins-agent
spec:
containers:
- name: jnlp
image: jenkins/inbound-agent:latest
- name: maven
image: maven:3.9.2
command: ["cat"]
tty: true
volumeMounts:
- name: maven-repo
mountPath: /root/.m2
- name: kubectl
image: lachlanevenson/k8s-kubectl
command: ["cat"]
tty: true
- name: docker
image: docker:latest
command: ["cat"]
tty: true
volumeMounts:
- name: docker-socket
mountPath: /var/run/docker.sock
volumes:
- name: docker-socket
hostPath:
path: /var/run/docker.sock
- name: maven-repo
persistentVolumeClaim:
claimName: maven-repo-pvc
'''
defaultContainer 'jnlp'
}
}
environment {
APP_NAME = 'my-microservice'
DOCKER_IMAGE = "username/${APP_NAME}"
K8S_NAMESPACE = 'default'
K8S_CREDENTIALS_ID = 'k8s-credentials'
DOCKER_CREDENTIALS_ID = 'docker-credentials'
SONARQUBE_CREDENTIALS_ID = 'sonarqube'
}
stages {
stage('Checkout') {
steps {
git branch: 'main', url: 'https://github.com/username/my-microservice.git'
}
}
stage('Build and Test') {
steps {
container('maven') {
sh 'mvn -Dmaven.repo.local=/root/.m2/repository clean test package'
}
}
}
stage('SonarQube Analysis') {
steps {
container('maven') {
withSonarQubeEnv('SonarQube') {
sh 'mvn sonar:sonar'
}
}
}
}
stage('Build and Push Docker Image') {
steps {
container('docker') {
script {
docker.withRegistry('https://index.docker.io/v1/', DOCKER_CREDENTIALS_ID) {
def customImage = docker.build("${DOCKER_IMAGE}:${BUILD_NUMBER}")
customImage.push()
customImage.push('latest')
}
}
}
}
}
stage('Deploy to K3s') {
steps {
container('kubectl') {
withKubeConfig([credentialsId: "${K8S_CREDENTIALS_ID}", namespace: "${K8S_NAMESPACE}"]) {
script {
sh """
kubectl apply -f kubernetes/
kubectl set image deployment/${APP_NAME} ${APP_NAME}=${DOCKER_IMAGE}:${BUILD_NUMBER} -n ${K8S_NAMESPACE}
kubectl rollout status deployment/${APP_NAME} -n ${K8S_NAMESPACE} --timeout=180s
"""
}
}
}
}
}
}
}
Jenkinsfile
Step 4: Explanation of Pipeline Stages
- Checkout: Pulls the latest code from the Git repository.
- Build and Test: Uses the
maven
container to compile and test the Java application. - SonarQube Analysis: Analyzes code quality with SonarQube.
- Build and Push Docker Image: Builds a Docker image for the application and pushes it to Docker Hub.
- Deploy to K3s: Uses
kubectl
to apply Kubernetes manifests, update the deployment image, and roll out the new version.
Step 5: Verifying the CI/CD Pipeline
After the pipeline runs successfully, verify:
- The Docker image is updated in Docker Hub.
- The application is redeployed in K3s, with pods running the latest Docker image.
Conclusion
Integrating Jenkins, Docker, and K3s for Java microservices CI/CD offers automated builds, testing, and deployments with high scalability. The Kubernetes plugin for Jenkins simplifies managing build agents by using K3s pods, optimizing both resource use and environment isolation. This setup not only reduces manual deployment efforts but also ensures a streamlined, automated pipeline for delivering quality software.